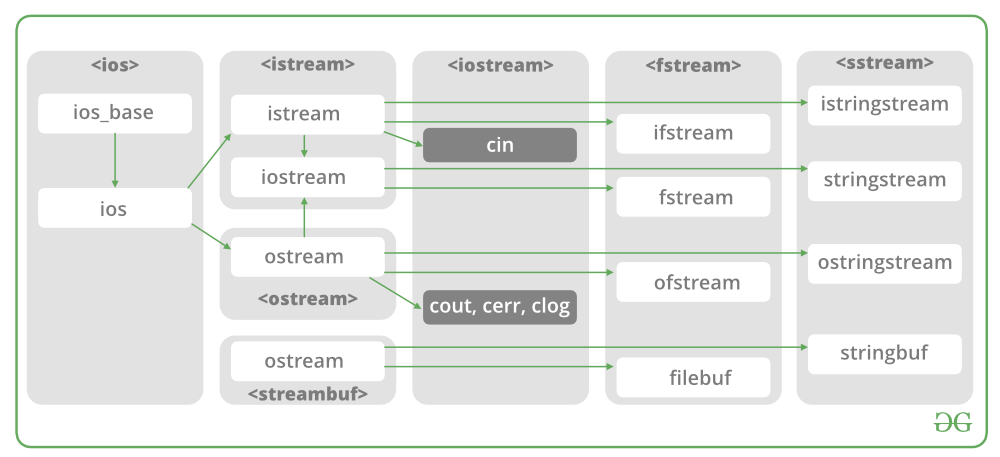
In C++, files are mainly dealt by using three classes fstream, ifstream, ofstream available in fstream headerfile.
ofstream: Stream class to write on files
ifstream: Stream class to read from files
fstream: Stream class to both read and write from/to files.
Now the first step to open the particular file for read or write operation. We can open file by
1. passing file name in constructor at the time of object creation
2. using the open method
For e.g.
Open File by using constructor
ifstream (const char* filename, ios_base::openmode mode = ios_base::in);
ifstream fin(filename, openmode) by default openmode = ios::in
ifstream fin(“filename”);Open File by using open method
Calling of default constructor
ifstream fin;fin.open(filename, openmode)
fin.open(“filename”);
Modes :
MEMBER CONSTANT | STANDS FOR | ACCESS |
---|---|---|
in * | input | File open for reading: the internal stream buffer supports input operations. |
out | output | File open for writing: the internal stream buffer supports output operations. |
binary | binary | Operations are performed in binary mode rather than text. |
ate | at end | The output position starts at the end of the file. |
app | append | All output operations happen at the end of the file, appending to its existing contents. |
trunc | truncate | Any contents that existed in the file before it is open are discarded. |
Default Open Modes :
ifstream | ios::in |
ofstream | ios::out |
fstream | ios::in | ios::out |
Below is the implementation by using fstream class.:
/* File Handling with C++ using ifstream & ofstream class object*/
/* To write the Content in File*/
/* Then to read the content of file*
#include <iostream>
/* fstream header file for ifstream, ofstream,
fstream classes */
#include <fstream>
using namespace std;
// Creation of ofstream class object
int main()
string line;
ofstream fout;
// by default ios::out mode, automatically deletes
// the content of file. To append the content, open in ios:app
// fout.open("sample.txt", ios::app)
fout.open("sample.txt");
// Execute a loop If file successfully opened
while (fout) {
// Read a Line from standard input
getline(cin, line);
// Press -1 to exit
if (line == "-1")
break;
// Write line in file
fout << line << endl;
}
// Close the File
fout.close();
// Creation of ifstream class object to read the file
ifstream fin;
// by default open mode = ios::in mode
fin.open("sample.txt");
// Execute a loop until EOF (End of File)
while (fin) {
// Read a Line from File
getline(fin, line);
// Print line in Console
cout << line << endl;
}
// Close the file
fin.close();
return 0;